此为TensorFlow框架官网学习笔记,原文为Getting Started With TensorFlow
The Computational Graph
tensorflow的核心程序由下面两个分离的部分组成
- Building the computational graph.
- Running the computational graph.
Tensorflow中的常量(constant)没有输入,输出一个内部存储的值,如下创建两个float类型的张量node1,node2如下:
输出的结果如下:
如上,并没有输出值3.0和4.0,如果要输出结果,需要使用session,如下,创建Session,然后输出节点值:
|
|
我们也可以通过联合Tensor来做一些复杂的操作,如下作加法:
输出的结果为:
TensorBoard中显示如下
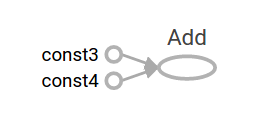
graph也可以被初始化接收一个外部输入,作为占位符(placeholder),表示随后提供一个值,如下:
|
|
在run中提供a和b的值
结果如下
同时也会提供变量(Variable)来修改参数,变量的定义如下W和b
Variable和constant的区别:constant可以被初始化,通过使用tf.constant,但初始化后的值不能被修改,而变量不能通过tf.Variable来初始化,初始化需要用统一的Tensorflow程序,如下:
变量通过assign来修改,如下,将W和b修改为-1和1,修改语句也需要在run中执行才能生效:
tf.train API
梯度下降
TensorFlow提供一个优化的方法,可以通过缓慢的修改变量来使得损失函数最小化,如下使用梯度下降来优化损失函数,这里在for循环中run了1000次,其中的train就执行了1000次,每次执行后都会修改W和b的值:
结果为:
评估函数
tf.estimator简化了机器学习的机制,包括运行训练模型,评估模型,管理数据集
定义如下模型:
训练数据及评估器:
这里用训练数据训练模型,然后用训练数据和测试数据评估模型
需要注意的是,这里的num_epochs如果有指定,表示每个元素产生num_epochs次,否则循环产生;shuffle表示是否打乱顺序,shuffle=False表示按顺序存储num_epochs次,否则乱序存储num_epochs次。
结果如下: